Need Sepolia ETH? Check out OP's recommended faucets 💦.
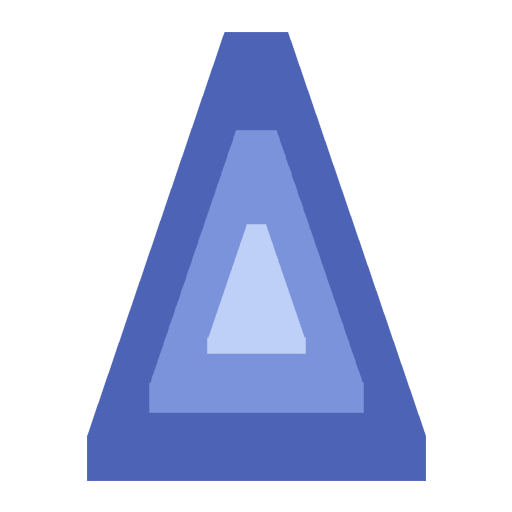
optimism-sepolia
/
#644
0x28705cdc961e78db9092879f657e4ff5b42c845f8a38810268c9de136dcda36b
Created:
03/12/2025 2:28:54 pm (21 days ago)
Resolver Contract:
Revocable Attestations:
Yes
Decoded Schema:
Non-standard schema
Raw Schema:
#! /usr/bin/env node
const { ethers } = require('ethers');
const { SchemaRegistry } = require('@ethereum-attestation-service/eas-sdk');
require('dotenv').config();
async function main() {
// Get configuration from environment variables
const providerUrl = process.env.OP_PROVIDER || 'https://sepolia.optimism.io';
const privateKey = process.env.PRIVATE_KEY;
if (!privateKey) {
console.error('ERROR: Private key not found. Make sure PRIVATE_KEY is set in your .env file.');
process.exit(1);
}
// Updated schema with uint64 types instead of string arrays for coordinates and weights
const schema =
'string userID, string portOrCompanyName, uint64[] portOrCompanyCoordinates,' +
'string actionType, string actionDate, uint64[] actionCoordinates, string collectorName,' +
'string[] incomingMaterials, uint64[] incomingWeightsKg, string[] incomingCodes,' +
'string[] outgoingMaterials, uint64[] outgoingWeightsKg, string[] outgoingCodes, ' +
'string productName, uint64 batchQuantity, uint64 weightPerItemKg';
console.log('Deploying schema to Optimism Sepolia...');
console.log(`Schema: ${schema}`);
try {
const timerStart = Date.now();
// Create provider and signer
const provider = new ethers.providers.JsonRpcProvider(providerUrl);
const wallet = new ethers.Wallet(privateKey, provider);
console.log(`Using wallet address: ${wallet.address}`);
// Schema Registry address for Optimism Sepolia
const schemaRegistryAddress = '0x4200000000000000000000000000000000000020';
// Create SchemaRegistry instance
const schemaRegistry = new SchemaRegistry(schemaRegistryAddress);
schemaRegistry.connect(wallet);
// Deploy the schema (no resolver, revocable)
const transaction = await schemaRegistry.register({
schema,
resolver: ethers.constants.AddressZero, // No resolver
revocable: true, // Make it revocable
});
console.log(`Transaction hash: ${transaction.hash}`);
console.log('Waiting for transaction confirmation...');
// Wait for transaction to be mined
const receipt = await transaction.wait();
// Get schema UID from transaction logs
const schemaUID = receipt.events[0].args.uid;
console.log(`Schema registration took ${Date.now() - timerStart} ms`);
console.log('Schema registered successfully!');
console.log('Schema UID:', schemaUID);
console.log(`View on Optimism Sepolia EAS Explorer: https://optimism-sepolia.easscan.org/schema/view/${schemaUID}`);
} catch (error) {
console.error('Failed to register schema:', error);
}
}
main();